09. Lab I: Solution
Solution: Build a Dog GraphQL API - Set Up
Below, we'll walk through each step of the lab and look at one potential way to implement the lab. Even if you get stuck, you should always first try to work through the lab without the solution before coming here, so that you can best learn the related skills and be ready for the project at the end of the course.
Step 1: Use Spring Initializr to bootstrap a simple project.
- Add the H2 Database, Spring Web Starter, and the Spring Data JPA dependencies before generating the project.
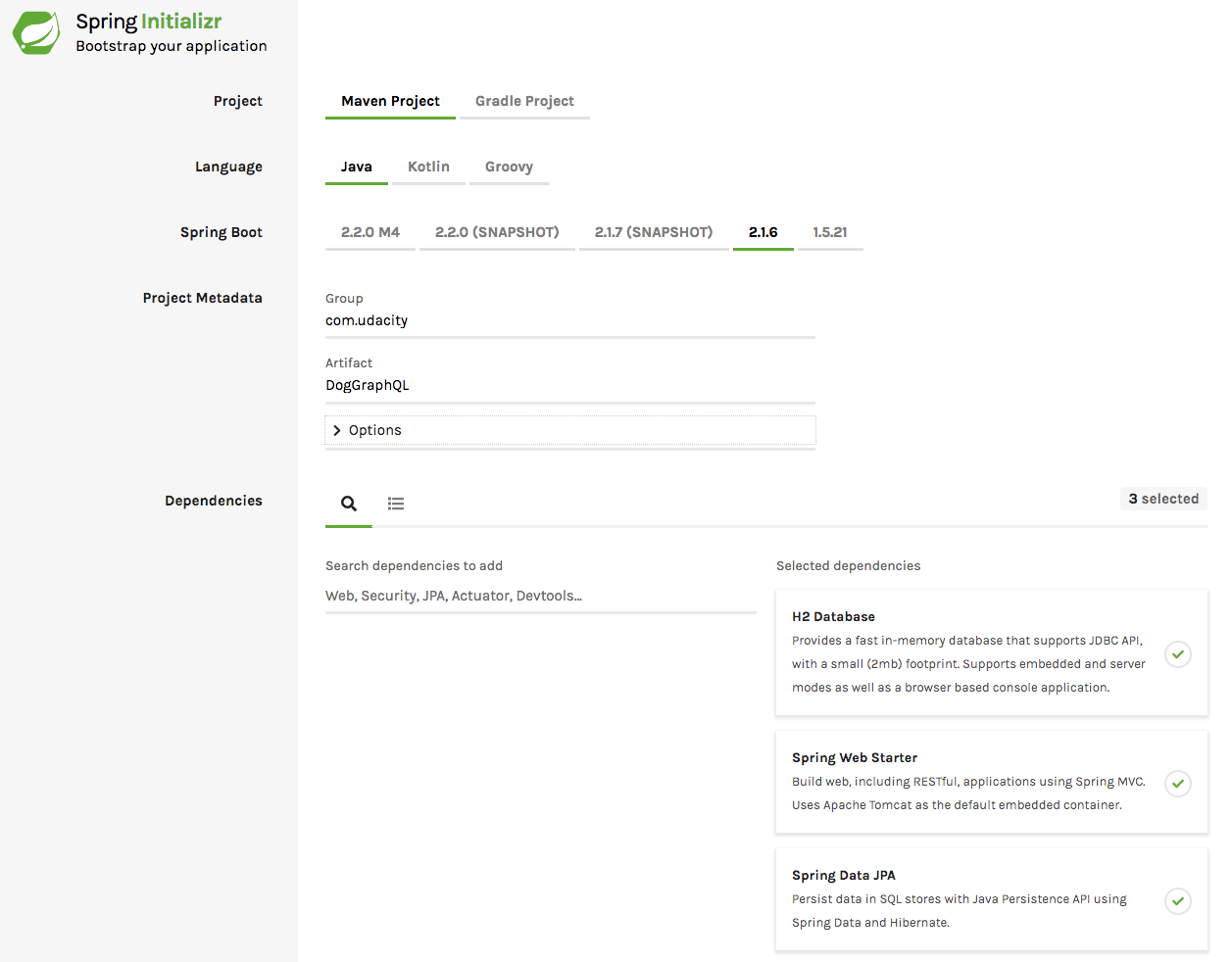
Select the correct dependencies for the project
Step 2: Add the necessary GraphQL dependencies.
- You will need to manually enter these in your Maven POM file.
- Set up the necessary H2 and GraphQL properties to
application.properties
at this time as well.
First, add the following dependencies in the pom.xml
file:
<dependency>
<groupId>com.graphql-java</groupId>
<artifactId>graphql-spring-boot-starter</artifactId>
<version>5.0.2</version>
</dependency>
<dependency>
<groupId>com.graphql-java</groupId>
<artifactId>graphql-java-tools</artifactId>
<version>5.2.4</version>
</dependency>
<dependency>
<groupId>com.graphql-java</groupId>
<artifactId>graphiql-spring-boot-starter</artifactId>
<version>5.0.2</version>
</dependency>
Then, head over to your application.properties
and add the following:
spring.h2.console.enabled=true
spring.h2.console.path=/h2
spring.datasource.url=jdbc:h2:mem:dogdata
graphql.servlet.mapping=/graphql
graphql.servlet.enabled=true
graphql.servlet.corsEnabled=true
graphiql.enabled=true
graphiql.endpoint=/graphql
graphiql.mapping=graphiql
Note that you don't necessarily have to use the exact same spring.datasource.url
, graphql.servlet.mapping
, graphiql.endpoint
, or graphiql.mapping
, but that will affect where you go down the road. However, graphql.servlet.mapping
and graphiql.endpoint
do need to match, as that is how GraphQL and GraphiQL will interact.